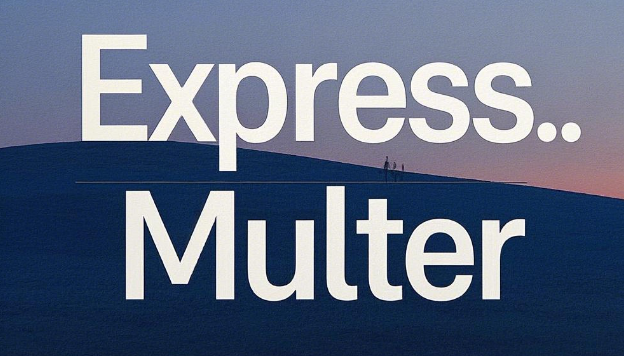
前言
Express
Express
是一个基于 Node.js 平台的极简、灵活的 Web 应用开发框架,它提供了一系列强大的特性来构建各种类型的 Web 服务和应用程序。
Multer
Multer
是一个用于处理 multipart/form-data
类型的文件上传的中间件,主要用于在 Express
应用中处理文件上传操作。
Cors
CORS
(Cross-Origin Resource Sharing)即跨域资源共享,是一种机制,它使用额外的 HTTP 头来告诉浏览器允许运行在一个源(域)上的 Web 应用程序访问来自不同源(域)的服务器上的指定资源。在前后端分离的开发环境中,特别是在使用 JavaScript 的 fetch
或 XMLHttpRequest
进行跨域请求时,CORS 是非常重要的。
演示例子
模块安装
在编写正式代码之前,先安装好 Express
、Multer
、Cors
这三个模块
npm install express multer cors
撰写代码
新建一个 app.js
文件将代码写入
const express = require('express');
const multer = require('multer');
const path = require('path');
const cors = require('cors'); // 导入 cors 中间件
const app = express();
const port = 3000;
// 使用 cors 中间件
app.use(cors());
// 配置存储引擎
const storage = multer.diskStorage({
destination: function (req, file, cb) {
// 设置文件存储路径、自己在根目录创建一个文件夹存放上传文件(这里以uploads为例)
cb(null, 'uploads/');
},
filename: function (req, file, cb) {
cb(null, Date.now() + path.extname(file.originalname));
}
});
// 初始化上传中间件
const upload = multer({ storage: storage });
// 单文件上传路由
app.post('/upload', upload.single('file'), (req, res) => {
if (!req.file) {
return res.status(400).send('未上传文件');
}
res.send('单文件上传成功!');
});
// 多文件上传路由
app.post('/upload-multiple', upload.array('files', 10), (req, res) => {
if (!req.files || req.files.length === 0) {
return res.status(400).send('未上传文件');
}
res.send('多文件上传成功');
});
// 启动服务器
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
运行代码
代码编写好后保存进行测试
node .\app.js
前端调用
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>上传案例</title>
</head>
<body>
<h2>单文件上传</h2>
<form id="singleFileForm" enctype="multipart/form-data">
<input type="file" name="file" id="singleFileInput">
<button type="submit">上传单文件</button>
</form>
<div id="singleFileResponse"></div>
<h2>多文件上传</h2>
<form id="multipleFilesForm" enctype="multipart/form-data">
<input type="file" name="files" id="multipleFilesInput" multiple>
<button type="submit">上传多个文件</button>
</form>
<div id="multipleFilesResponse"></div>
<script>
// 处理单文件上传的表单提交
document.getElementById('singleFileForm').addEventListener('submit', function(event) {
event.preventDefault();
const fileInput = document.getElementById('singleFileInput');
const file = fileInput.files[0];
const formData = new FormData();
formData.append('file', file);
fetch('http://localhost:3000/upload', {
method: 'POST',
body: formData
})
.then(response => response.text())
.then(data => {
document.getElementById('singleFileResponse').innerText = data;
})
.catch(error => {
document.getElementById('singleFileResponse').innerText = '错误: ' + error;
});
});
// 处理多文件上传的表单提交
document.getElementById('multipleFilesForm').addEventListener('submit', function(event) {
event.preventDefault();
const fileInputs = document.getElementById('multipleFilesInput');
const formData = new FormData();
for (let i = 0; i < fileInputs.files.length; i++) {
formData.append('files', fileInputs.files[i]);
}
fetch('http://localhost:3000/upload-multiple', {
method: 'POST',
body: formData
})
.then(response => response.text())
.then(data => {
document.getElementById('multipleFilesResponse').innerText = data;
})
.catch(error => {
document.getElementById('multipleFilesResponse').innerText = '错误: ' + error;
});
});
</script>
</body>
</html>
如图所示:
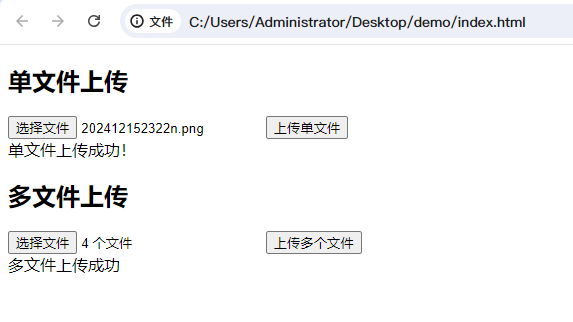
结语
一个基于 Node.js
和 Express
以及cors
的文件上传功能实现案例就完成啦,本案例涵盖后端服务器代码及前端页面调用。后端使用 Multer
中间件处理文件上传和存储引擎配置,完成单文件与多文件上传路由处理;采用 cors
中间件解决跨域问题,控制不同源请求,保障服务器安全。前端利用 HTML
表单和 JavaScript
的 fetch API
与后端交互。还提供了启动本地服务器、配置特定源、前端设置代理等跨域解决方案。此案例为文件上传功能开发提供全面参考,可作为拓展优化构建丰富文件处理应用的基础。
1. 本栈资源大多存储在云盘,如发现链接失效,请联系我们,我们会第一时间更新。
2. 本栈内资源或文章大多收集自网络,请勿应用于商业用途,如有其它需求,请购买正版支持作者,谢谢!
3. 本栈收集的资源仅供内部学习研究软件设计思想和原理使用,学习研究后请自觉删除,请勿传播,因未及时删除所造成的任何后果责任自负。
4. 若您认为「UPKG.CN」发布的内容若侵犯到您的权益,请联系站长邮箱: admin@upkg.cn 进行删除处理。
暂无评论内容